If you use jquery events like below:
Sometimes it can be useful to check what events are assigned to what elements (imagine you have much bigger html application with many external javascripts and libraries)<!doctype html> <head> <meta charset="utf-8"> <script src="./jquery-1.10.2.js"></script> <script> $(function() { $('#link').click(function(e) { console.log('klik jquery', e); return false; }); }); $(function() { $('#link').change(function(e) { console.log('change jquery', e); return false; }); }); </script> </head> <body> <a id="link" href="" >Set currency</a> <br /> <select id="ddl"> <option value="None">None</option> <option value="GBP">Sterling</option> <option value="EUR">Euro</option> </select> </body>
jQuery uses .data function to store events with key 'events'.
$._data(element, 'events') or $('#elem').data('events') in older versions of jquery
Result:
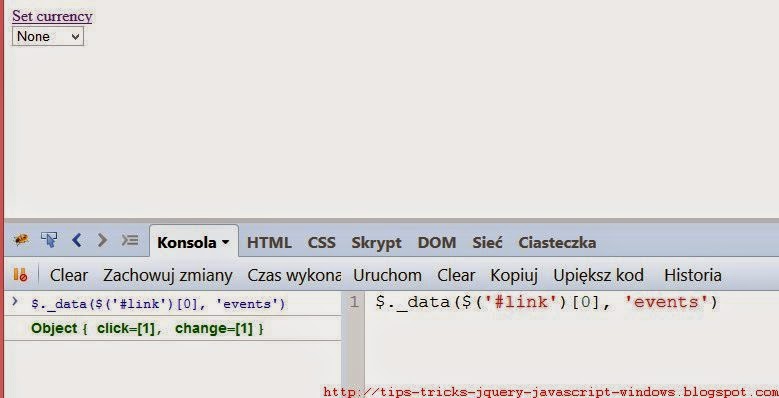
Or even simpler - select element in firebug
and then you can write:
$._data($0, 'events')
$0 - this is last selected element in firebug or chrome developer tools
You can expand returned object to see what events are assigned
In firebug you can click on "function" to see the source of this function (or try clicking right mouse button)
Is it possible to see all events for all elements? Yes, with simple script :)
Result:clear(); $('*').each(function() { if ($._data(this, 'events')) { console.log(this); $.each($._data(this, 'events'), function() { console.log("***"+this[0].type); console.log(this[0].handler.toString()); }); }